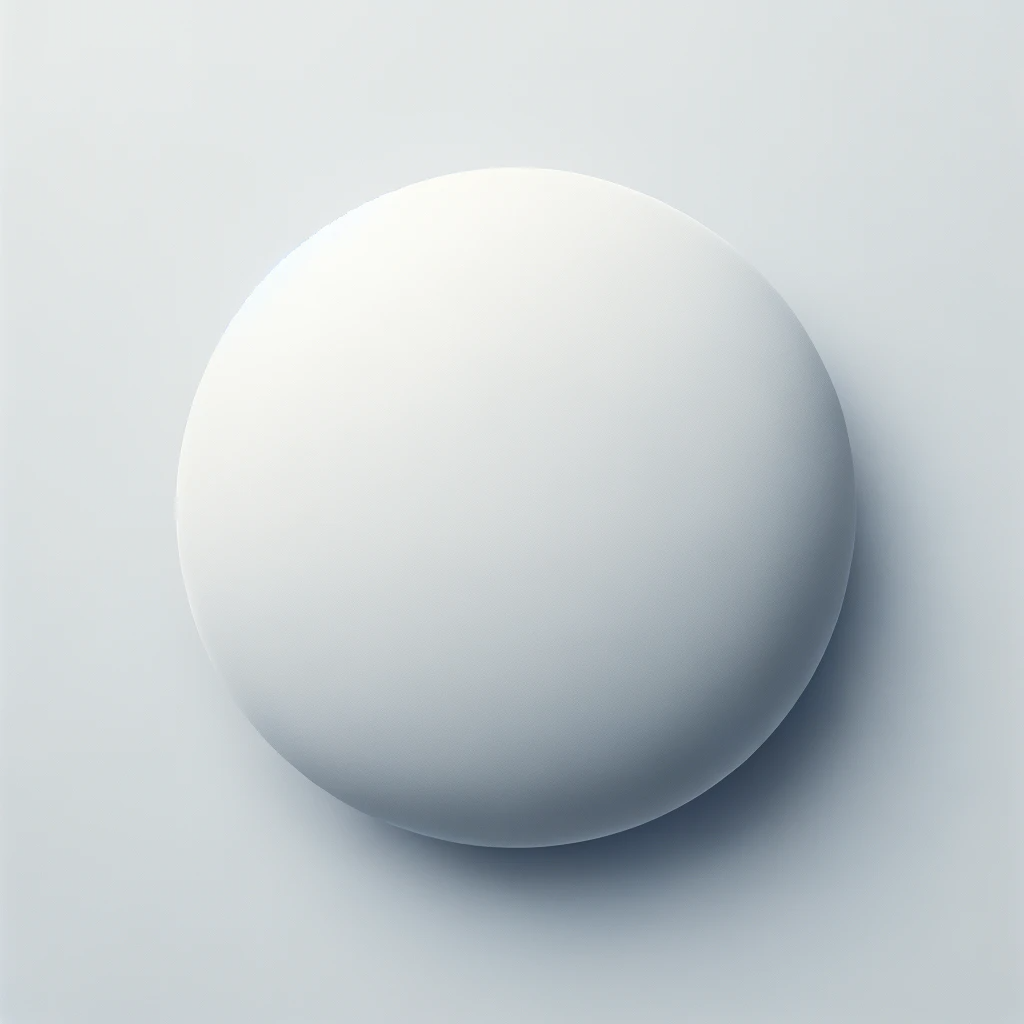
The above will modify the existing list. If you wish to create a new list, you need to return the new list: def fixlist(lst, st): new_list = lst+[st] return new_list. The above will create a new list with lst and st, and returns it. Try to fix your code now, if that still fails, edit the question with your attempts.Evaluate an expression node or a string containing only a Python literal or container display. The string or node provided may only consist of the following Python literal structures: strings, bytes, numbers, tuples, lists, dicts, sets, booleans, None and Ellipsis.What needs to be done is iterate through the arguments passed through *args and check if the type of argument, if it's list then you have passed a list i.e. average([1,3,5,7]) else it will be integer when integers are passed i.e. average(1,3,5,7). So following piece of code will work :-Python is a versatile programming language that is widely used for various applications, from web development to data analysis. One of the best ways to learn and practice Python is...I am a beginner in python and met with a requirement to declare/create some lists dynamically for in python script. I need something like to create 4 list objects like depth_1,depth_2,depth_3,depth_4 on giving an input of 4.Like In Python, you can have a List of Dictionaries. You already know that elements of the Python List could be objects of any type. In this tutorial, we will learn how to create a list of dictionaries, how to access them, how to append a dictionary to list and how to modify them. The problem is not that idx is a list but probably that x is an array - old_list must contain a list as an element. You need to reference the index, and not the item itself: [old_list[x] for x in range(len(old_list)) if idx[x] == 3] here's a minimal example:In Python, list slicing is a common practice and it is the most used technique for programmers to solve efficient problems. Consider a Python list, in order to access a range of elements in …In Python, lists are ordered collections of items that allow for easy use of a set of data. List values are placed in between square brackets [ ] ...Create a Python List. We create a list by placing elements inside square brackets [], …Evaluate an expression node or a string containing only a Python literal or container display. The string or node provided may only consist of the following Python literal structures: strings, bytes, numbers, tuples, lists, dicts, sets, booleans, None and Ellipsis.I would like to create an Array from two Arrays but I do not want to create this new Array, with append() or extend(). Input arrays have the same number of rows and columns: listone = [1,2,3] listt...@Luca: If you're concerned about performance then use itertools.product, since it's written in C, and C code runs significantly faster than Python code.And as others have said, it's often better to use a generator that yields the items one by one rather than building a RAM-hogging list, unless you actually need the whole collection of items as a …Creating a List in Python. Lists in Python can be created by just placing the sequence inside …Creating Python Lists. Let’s now take a look at how we can create Python lists. To create an empty list, you have two different options: Using the list() function; Using empty square brackets, [] Let’s take a look at what this looks like: # Creating an Empty List in Python empty_list1 = list() empty_list2 = [] We can also create lists with ...A Python linked list is an abstract data type in Python that allows users to organize information in nodes, which then link to another node in the list. This makes it easier to insert and remove information without changing the index of other items in the list. You want to insert items easily in between other items. In Python, you can have a List of Dictionaries. You already know that elements of the Python List could be objects of any type. In this tutorial, we will learn how to create a list of dictionaries, how to access them, how to append a dictionary to list and how to modify them. According to the Smithsonian National Zoological Park, the Burmese python is the sixth largest snake in the world, and it can weigh as much as 100 pounds. The python can grow as mu...Create a Python List. We create a list by placing elements inside square brackets [], …Pseudo-Code. def main(): create an empty list value = getInput() while value isnt zero: add value to the list value = getInput() printOutput(list) def getInput(): prompt the user for a value make sure that the value is an int (convert to int) return the number def printOutput(list): print out the number of input values print out the individual input values …Classification: Python is a high-level object-oriented scripting language. Usage: Back-end web developers use Python to create web …Dec 4, 2012 · a = ' ' + string.ascii_uppercase. # if start > end, then start from the back. direction = 1 if start < end else -1. # Get the substring of the alphabet: # The `+ direction` makes sure that the end character is inclusive; we. # always need to go one *further*, so when starting from the back, we. # need to substract one. Opens the CSV Defined by the user, splits the file into different Predefined "pools" and remakes them again into their own files, with proper headers. My only problem is I want to change the Pool list from a static to a variable; and having some issues. The pool list is in the CSV it self, in column 2. and can be duplicated.When it comes to planning a wedding, one of the most important tasks is creating a bridal registry list. This list will help your guests know what items you need for your special d...To create a dictionary we can use the built in dict function for Mapping Types as per the manual the following methods are supported. dict(one=1, two=2) dict({'one': 1, 'two': 2}) dict(zip(('one', 'two'), (1, 2))) dict([['two', 2], ['one', 1]]) The last option suggests that we supply a list of lists with 2 values or (key, value) tuples, … Creating a list in python is very simple. You can create an empty list L like this. # This is a python list L that has no items stored. L = [] Lists in python are declared using square brackets. What goes inside these brackets is a comma separated list of items. If no items are provided, an empty list is created. Note that in the third case, since lists are referred to by reference, changing one instance of ['x','y'] in the list will change all of them, since they all refer to the same list. To avoid referencing the same item, you can use a comprehension instead to create new objects for each list element: L = [['x','y'] for i in range(20)]The other answers already mention that lists in python are dynamic, so you can specify an empty list and append to it. Also, other operations are supported, such as insert list.insert(i, x) (inserts an element at a given position), which allows you place an element anywhere in the array, not just at the end.To create a stack in Python you can use a class with a single attribute of type list. The elements of the stack are stored in the list using the push method and are retrieved using the pop method. Additional methods allow to get the size of the stack and the value of the element at the top of the stack.Mar 25, 2022 · List of Lists Using the append() Method in Python. We can also create a list of lists using the append() method in python. The append() method, when invoked on a list, takes an object as input and appends it to the end of the list. To create a list of lists using the append() method, we will first create a The try statement works as follows. First, the try clause (the statement (s) between the try and except keywords) is executed. If no exception occurs, the …I want to create a list of times in the format 00:00 to 23:45. I think this can be done using a for loop, but I am unsure of how to do it. I don't care about the type of the values in the list as long as the correct format of 00:00 is used. Right now I just instantiate the list with the set values, code shown below. Thank you for your help ...A fixed-size list is a list that has a predefined number of elements and does not change in size. In Python, while lists are inherently dynamic, we can simulate ...In Python, lists are ordered collections of items that allow for easy use of a set of data. List values are placed in between square brackets [ ] ...When it comes to hiring school cleaners, one of the most important aspects is creating an effective job description. A well-written job description helps attract qualified candidat...I would like to set a list of hosts (IPs) on which my Flask server can listen on so that if the main Ethernet port blows up there is the other one that can …Aban 24, 1398 AP ... Surround the sequence of data points with brackets. Now let's create five lists, one for each row in our dataset: row_1 = [ ...The LinkedList class we eventually build will be a list of Node s. class Node: def __init__(self, val): self.val = val self.next = None def set_next(self, node): self.next = node def __repr__(self): return self.val. Each node has a val data member (the information it stores) and a next data member. The next data member just points to the next ...Yes, creating a list of lists is indeed the easiest and best solution. I do favor teaching new Python users about list comprehensions, since they exhibit a clean, expression-oriented style that is increasingly popular among Pythonistas. – Fred Foo. Jul 11, 2011 at 12:12. 1.from Tkinter import *. master = Tk() variable = StringVar(master) variable.set("one") # default value. w = OptionMenu(master, variable, "one", "two", "three") w.pack() mainloop() More information (including the script above) can be found here. Creating an OptionMenu of the months from a list would be as …For creating a list of lists of zeroes (when numpy isn't an option) this was what i came up with: p_ij = [[0] * k for i in range(k)] that way it wouldn't share an ID and when you made an assignment such as p_ij[2][3] it wouldn't …Python is a versatile programming language that is widely used for various applications, from web development to data analysis. One of the best ways to learn and practice Python is...When it comes to planning a wedding, one of the most important tasks is creating a bridal registry list. This list will help your guests know what items you need for your special d...Python is a versatile programming language that is widely used for various applications, from web development to data analysis. One of the best ways to learn and practice Python is...from Tkinter import *. master = Tk() variable = StringVar(master) variable.set("one") # default value. w = OptionMenu(master, variable, "one", "two", "three") w.pack() mainloop() More information (including the script above) can be found here. Creating an OptionMenu of the months from a list would be as …To create a list of lists in python, you can use the square brackets to store all the inner lists. For instance, if you have 5 lists and you want to create a …Set, a term in mathematics for a sequence consisting of distinct languages is also extended in its language by Python and can easily be made … If we compare the runtimes, among random list generators, random.choices is the fastest no matter the size of the list to be created. However, for larger lists/arrays, numpy options are much faster. So for example, if you're creating a random list/array to assign to a pandas DataFrame column, then using np.random.randint is the fastest option. A list is generated in Python programming by putting all of the items (elements) inside square brackets [], separated by commas. It can include an unlimited number of elements of various data types (integer, float, string, etc.). Python Lists can also be created using the built-in list () method. Example.If we compare the runtimes, among random list generators, random.choices is the fastest no matter the size of the list to be created. However, for larger lists/arrays, numpy options are much faster. So for example, if you're creating a random list/array to assign to a pandas DataFrame column, then using …Sep 30, 2022 · A Python linked list is an abstract data type in Python that allows users to organize information in nodes, which then link to another node in the list. This makes it easier to insert and remove information without changing the index of other items in the list. You want to insert items easily in between other items. Methods in contrast act on objects. Python offers the following list functions: sort (): Sorts the list in ascending order. type (list): It returns the class type of an object. append (): Adds a single element to a list. extend (): Adds multiple elements to a list. We can join two lists with the + operator. It adds integers and floats but concatenates strings and lists. ... We can make a list repeat by multiplying it by an ... The list () function creates a list object. A list object is a collection which is ordered and changeable. Read more about list in the chapter: Python Lists. How to Create a List in Python. To create a list in Python, we use square brackets ( [] ). Here's what a list looks like: ListName = [ListItem, …Use list comprehension in python. Since you want 16 in the list too.. Use x2+1. Range function excludes the higher limit in the function. list=[x for x in range(x1, x2+1)] Share. Improve this answer. Follow ... Creating a list or range with all numbers up to a certain variable. 0.May 6, 2023 · Create a list of floats in Python using map () Function. Another approach to create a list of floats is by using the map () function. This function applies a given function to each item of an iterable (e.g., list, tuple) and returns a new iterable (e.g., a list or a map object). Creating a Histogram in Python with Matplotlib. To create a histogram in Python using Matplotlib, you can use the hist() function. This hist function takes a number of arguments, the key one being the bins argument, which specifies the number of equal-width bins in the range. Tip!I want to create a series of lists with unique names inside a for-loop and use the index to create the liste names. Here is what I want to do x = [100,2,300,4,75] for i in x: list_i=[] I want...In Python, creating a list of lists using a for loop involves iterating over a range or an existing iterable and appending lists to the main list. This approach allows for the dynamic generation of nested lists based on a specific pattern or condition, providing flexibility in list construction. In this article, we will …What needs to be done is iterate through the arguments passed through *args and check if the type of argument, if it's list then you have passed a list i.e. average([1,3,5,7]) else it will be integer when integers are passed i.e. average(1,3,5,7). So following piece of code will work :-There’s an element of confusion regarding the term “lists of lists” in Python. I wrote this most comprehensive tutorial on list of lists in the world to remove all those confusions by beginners in the Python programming language.. This multi-modal tutorial consists of: Source code to copy&paste in your own projects.; Interactive code you can …Creating Lists Faster. Image by Leslie Zambrano from Pixabay. In Python, List is no doubt the most commonly used data structure for its flexibility. ... We know that … A list is generated in Python programming by putting all of the items (elements) inside square brackets [], separated by commas. It can include an unlimited number of elements of various data types (integer, float, string, etc.). Python Lists can also be created using the built-in list () method. Example. Method 3: Create a matrix in Python using matrix () function. Use the numpy.matrix () function to create a matrix in Python. We can pass a list of lists or a string representation of the matrix to this function. Example: Let’s create a 2*2 matrix in Python. import numpy as np.We can join two lists with the + operator. It adds integers and floats but concatenates strings and lists. ... We can make a list repeat by multiplying it by an ...Mar 12, 2024 · Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () function to it, forming a 2D list. The resulting structure is then printed using the `print` statement. Python. Here, you instantiate an empty list, squares.Then, you use a for loop to iterate over range(10).Finally, you multiply each number by itself and append the result to the end of the list.. Work With map Objects. For an alternative approach that’s based in functional programming, you can use map().You pass in a function and an iterable, and …It depends on how the list particularly was implemented. For example, if you already have a list that you pass as an argument, the upper bound for creating a copy or appending a list at the end of it might be O(1).But, for the general case where you append n elements to a list, assuming you have a …YouTube has become a go-to platform for live streaming, with millions of viewers tuning in every day to watch their favorite content creators. If you’re looking to expand your audi...Python's syntax for declaring an empty list is names = [], not names []. Once you've declared the list and put some items into it - e.g. names.append ('John Smith') - you can then access items in the list using the names [] syntax - names [0] for the first element in the list, for example. If you're having this kind of trouble with basic ...Lists and tuples are arguably Python’s most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here’s what you’ll learn in this tutorial: You’ll cover the important characteristics of lists and tuples. You’ll learn how to define them and how to manipulate them. If you haven't used generators before, note that you loop through them as if they were a list, such as this: # a generator returned instead of list. my_combinations = all_combinations([1,2,3]) # this would also work if `my_combinations` were a list. for c in my_combinations: print "Combo", c. """. Prints: A list is generated in Python programming by putting all of the items (elements) inside square brackets [], separated by commas. It can include an unlimited number of elements of various data types (integer, float, string, etc.). Python Lists can also be created using the built-in list () method. Example.Creating dynamic variables is rarely a good idea and it might affects performance. You can always use dictionary instead as it would be more appropriate: lists = {} lists["list_" + str(i)] = [] lists["list_" + str(i)].append(somevalue) Look here for some more explanation: Creating a list based on value stored in variable in pythonPython Convert String to List using re.findall () method. This task can be performed using regular expression. We can use the pattern to match all the alphabet and make a list with all the matched elements. Python3. import re. def Convert (string): return re.findall (' [a-zA-Z]', string) str1="ABCD".Python For Loops. A for loop is used for iterating over a sequence (that is either a list, a tuple, a dictionary, a set, or a string).. This is less like the for keyword in other programming languages, and works more like an iterator method as found in other object-orientated programming languages.. With the for loop we can execute a …. @loved.by.Jesus: Yeah, they added optimizatiPython is a powerful and versatile programming langu I would like to set a list of hosts (IPs) on which my Flask server can listen on so that if the main Ethernet port blows up there is the other one that can …Jul 10, 2019 · 3) Appending two lists to a single list. Usually appending two lists can be done by using append( ) method, but appending can also be done by using a ‘+’ (here + doesn't mean addition) whereas + can be used to add or merge two lists into a single list as shown below. How to create a Python list. Let’s start by creating a list: my_ Dec 27, 2023 · Python List comprehension provides a much more short syntax for creating a new list based on the values of an existing list. List Comprehension in Python Example. Here is an example of using list comprehension to find the square of the number in Python. Creating a List in Python. Lists in Python can be created by just placing the sequence inside … To start, here is a template that you may use to create a list in Pyt...
Continue Reading