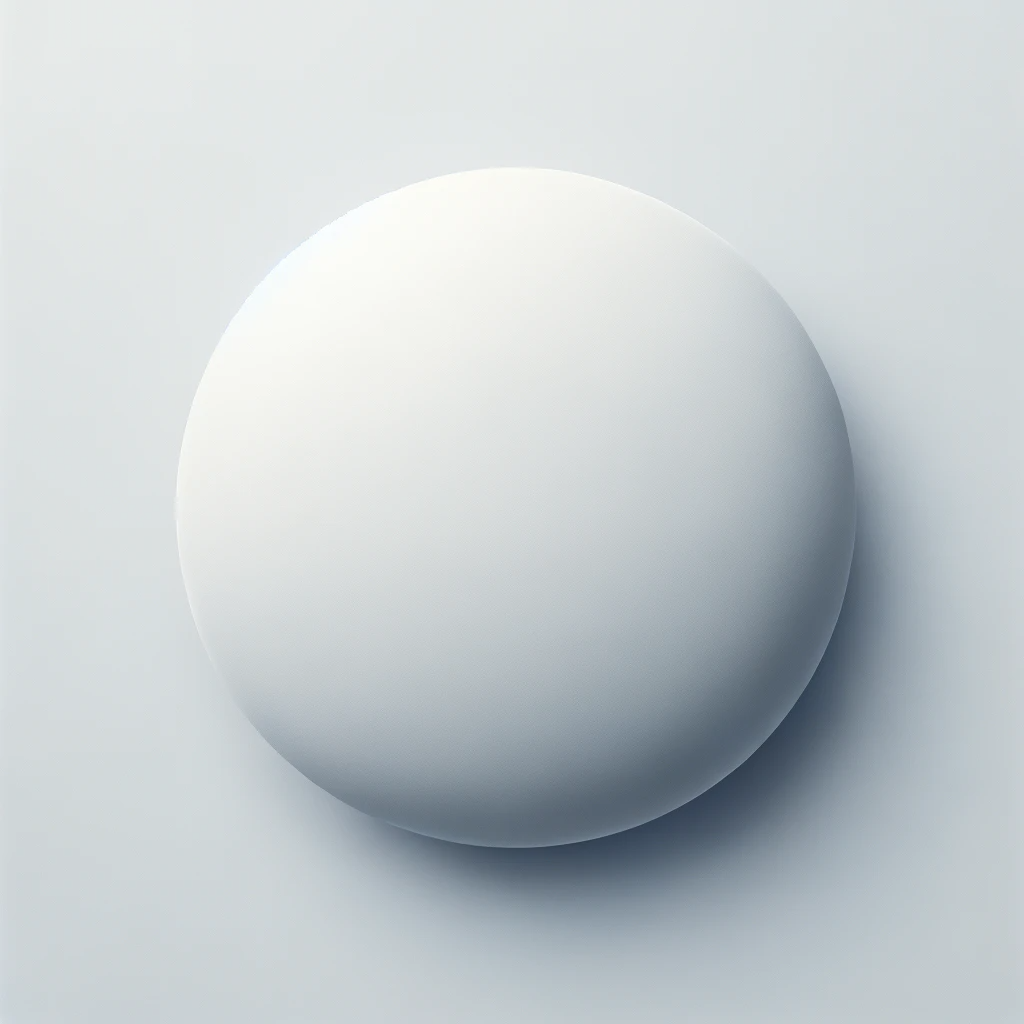
In python, as far as I know, there are at least 3 to 4 ways to create and initialize lists of a given size: Simple loop with append:. my_list = [] for i in range(50): my_list.append(0) Learn three ways to create lists in Python: using loops, map(), and list comprehensions. See examples of how to create lists of elements, calculate the …To create a dictionary we can use the built in dict function for Mapping Types as per the manual the following methods are supported. dict(one=1, two=2) dict({'one': 1, 'two': 2}) dict(zip(('one', 'two'), (1, 2))) dict([['two', 2], ['one', 1]]) The last option suggests that we supply a list of lists with 2 values or (key, value) tuples, so we ...Apr 13, 2023 ... The list in Python is one of the fundamental data structures used to store multiple items in a single variable. You can create a list by ...You can create a Python list called grocery_list to keep track of all the items you need to buy. Each item, such as "apples," "bananas," or "milk," is like an element in your list. Here's what a simple grocery list might look like in Python: grocery_list = ["apples", "bananas", "milk"]Let’s see all the different ways to iterate over a list in Python and the performance comparison between them. Using for loop. Using for loop and range () Using a while loop. Using list comprehension. Using enumerate () method. Using the iter function and the next function. Using the map () function. Using zip () Function.A Python list is a convenient way of storing information altogether rather than individually. As opposed to data types such as int, bool, float, str, a list is a compound data type where you can group values together. In Python, it would be inconvenient to create a new python variable for each data point you collected. A Python list is a convenient way of storing information altogether rather than individually. As opposed to data types such as int, bool, float, str, a list is a compound data type where you can group values together. In Python, it would be inconvenient to create a new python variable for each data point you collected. In Python, creating a list of lists using a for loop involves iterating over a range or an existing iterable and appending lists to the main list. This approach allows for the dynamic generation of nested lists based on a specific pattern or condition, providing flexibility in list construction. In this article, we will explore four different ...As the docs state, list comprehensions are used to create a list using a for-loop and has the general structure: [expression for item in iterable (0 or more if/for clauses)] It can create either: a list by evaluating expression in iteration, or; a subsequence from iterable if an if statement follows the initial for statementAug 20, 2023 ... Create an empty list ... An empty list is created as follows. You can get the number of elements in a list using the built-in len() function.2 days ago · List comprehensions provide a concise way to create lists. Common applications are to make new lists where each element is the result of some operations applied to each member of another sequence or iterable, or to create a subsequence of those elements that satisfy a certain condition. For example, assume we want to create a list of squares, like: Create your own server using Python, PHP, React.js, Node.js, Java, C#, etc. How To's. Large collection of code snippets for HTML, CSS and JavaScript. ... List Methods. Python has a set of built-in methods that you can use on lists. Method Description; append() Adds an element at the end of the list:Apr 9, 2021 · Python list is an ordered sequence of items. In this article you will learn the different methods of creating a list, adding, modifying, and deleting elements in the list. Also, learn how to iterate the list and access the elements in the list in detail. Nested Lists and List Comprehension are also discussed in detail with examples. for j in range(0, n): number = 2 ** j. myList.append(number) return myList. I want this code to return the powers of 2 based upon the first n powers of 2. So for example, if I enter in 4, I want it to return [2,4,6,8,16]. Right now, the code returns [1,2,4,8] if I enter in 4. I think it's my range that's messing up the code. python.Python Program to Write a List to a File in Python Using a Loop Imagine you are building an application that has to persist a list of items after its execution. This might be required, for example, if you store data in a file every time you run your application and that data can be available for later use the next time you run your application.W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.Feb 8, 2024 · The below code initializes an empty list called listOfList and, using a nested for loop with the append () method generates a list of lists. Each inner list corresponds to a row, and the elements in each row are integers from 0 to the row number. The final result is displayed by printing each inner list within listOfList. Python. listOfList = [] First, an initialization step: Create an item M. Create a list L and add M to L. Second, loop through the following: Create a new item by modifying the last item added to L. Add the new item to L. As a simple example, say I want to create a list of lists where the nth list contains the numbers from 1 to n. The list () function creates a list object. A list object is a collection which is ordered and changeable. Read more about list in the chapter: Python Lists. Nov 29, 2023 · Below are the ways by which we can use list() function in Python: To create a list from a string; To create a list from a tuple; To create a list from set and dictionary; Taking user input as a list; Example 1: Using list() to Create a List from a String. In this example, we are using list() function to create a Python list from a string. However, in some cases, you’ll want to convert the ranges you create into Python lists. Thankfully, this is made easy by using the list() constructor function. You can simply pass the range into the list() function, which creates a list out of the range. Let’s see what this looks like: # Converting a Python Range Into a List list_of_values ...To create a list of numbers from 1 to N in Python using the range () function you have to pass two arguments to range (): “start” equal to 1 and “stop” equal to N+1. Use the list () function to convert the output of the range () function into a list and obtain numbers in the specified range.Create lists of data. One of the foremost tasks in a batch processing script is cataloging the available data so it can iterate through the data during processing. ArcPy has a number of functions built specifically for creating such lists. The result of each of these functions is a list, which is a list of values.Aug 21, 2023 · The ` tolist() ` function, a method provided by NumPy arrays, is then used to convert the NumPy array into a standard Python list, and the resulting list is stored in the variable `list_from_numpy`. Finally, the content of `list_from_numpy` is printed, displaying the converted Python list containing the same elements as the original NumPy array. Python programming has gained immense popularity in recent years due to its simplicity and versatility. Whether you are a beginner or an experienced developer, learning Python can ...Jun 17, 2020 · The list is one of the most useful data-type in python. We can add values of all types like integers, string, float in a single list. List initialization can be done using square brackets []. Below is an example of a 1d list and 2d list. As we cannot use 1d list in every use case so python 2d list is used. Also, known as lists inside a list or ... Example 1: Create lists from string, tuple, and list. # empty list print(list()) # vowel string . vowel_string = 'aeiou' print (list(vowel_string)) # vowel tuple . vowel_tuple …May 6, 2023 · Create a list of floats in Python using map () Function. Another approach to create a list of floats is by using the map () function. This function applies a given function to each item of an iterable (e.g., list, tuple) and returns a new iterable (e.g., a list or a map object). How to create, access dictionaries in list, and then update or delete key:value pairs, or append dictionary to the list. Python Examples. Tutorials Exercises ... Create a List of Dictionaries in Python. In the following program, we create a list of length 3, …Objective: Create a list with data and make use of that list by passing it to a function. The vertices of a star centered the middle of a small drawing area are ...Values of a: [] Type of a: <class 'list'> Size of a: 0 Python Append to Empty List. In Python, we can create an empty list and then later append items to it, using the Python append() function. Example: In this example, we will first create an empty list in Python using the square brackets and then appended the items to it.In this example, the list comprehension creates a new list containing the integer value 1 repeated 5 times. The _ in the list comprehension …In your example, x is a list containing three elements (integers). With append, you add a new element. By appending y, you are adding a list as a fourth element (3 integers, one list). If you want to create a list of lists, tread x and y as elements for that, and combine them in a list: x = [1,2,3] y = [4,5,6] list_of_lists = [x,y]Data Structures — Python 3.12.2 documentation. 5. Data Structures ¶. This chapter describes some things you’ve learned about already in more detail, and adds some new things as well. 5.1. More on Lists ¶. The list data type has some more methods. Here are all of the methods of list objects:How to Create a List in Python. You can create a list in Python by separating the elements with commas and using square brackets []. Let's create …Create your own server using Python, PHP, React.js, Node.js, Java, C#, etc. How To's. Large collection of code snippets for HTML, CSS and JavaScript. ... List Methods. Python has a set of built-in methods that you can use on lists. Method Description; append() Adds an element at the end of the list:You're technically trying to index an uninitialized array. You have to first initialize the outer list with lists before adding items; Python calls this "list comprehension". # Creates a list containing 5 lists, each of 8 items, all set to 0 w, h = 8, 5 Matrix = [[0 for x in range(w)] for y in range(h)] #You can now add items to the list:Learn how to create, access, update, and manipulate lists in Python with this tutorial. Discover the key features, operations, and use cases of lists, …How to Create a Dictionary in Python. A dictionary in Python is made up of key-value pairs. In the two sections that follow you will see two ways of creating a dictionary. The first way is by using a set of curly braces, {}, and the second way is by using the built-in dict () function.Nov 29, 2023 · Below are the ways by which we can use list() function in Python: To create a list from a string; To create a list from a tuple; To create a list from set and dictionary; Taking user input as a list; Example 1: Using list() to Create a List from a String. In this example, we are using list() function to create a Python list from a string. Dec 27, 2023 · Python List comprehension provides a much more short syntax for creating a new list based on the values of an existing list. List Comprehension in Python Example. Here is an example of using list comprehension to find the square of the number in Python. Create Lists using Python. To create a list in Python, you place your data items in a comma-separated list, enclosed between two square brackets or “[].” Lists are “ordered” in the sense that, once that list is created, the order of the items does not change.This is the standard method of creating lists in Python. To create an empty list, just use a pair of square brackets with nothing inside: empty_list = [] To create a list with initial elements, put the element values inside the square brackets separated by commas: numbers = …Data Structures — Python 3.12.2 documentation. 5. Data Structures ¶. This chapter describes some things you’ve learned about already in more detail, and adds some new things as well. 5.1. More on Lists ¶. The list data type has some more methods. Here are all of the methods of list objects:The syntax for the “not equal” operator is != in the Python programming language. This operator is most often used in the test condition of an “if” or “while” statement. The test c...Example 1: Create lists from string, tuple, and list. # empty list print(list()) # vowel string . vowel_string = 'aeiou' print (list(vowel_string)) # vowel tuple . vowel_tuple …I have a text file called "test", and I would like to create a list in Python and print it. I have the following code, but it does not print a list of words; it prints the whole document in one lin... In python, as far as I know, there are at least 3 to 4 ways to create and initialize lists of a given size: Simple loop with append:. my_list = [] for i in range(50): my_list.append(0) 10. You can call it like list ( [iterable]), so the optional needs to be iterable, which float isn't. iterable may be either a sequence, a container that supports iteration, or an iterator object. The direct definition as list would work …Python has become one of the most popular programming languages in recent years. Whether you are a beginner or an experienced developer, there are numerous online courses available...Sep 25, 2022 · list2 = list (dict) print (list2) [‘name’, ‘age’, ‘gender’] Converting a dictionary using list (dict) will extract all its keys and create a list. That is why we have the output [‘name’,’age’,’gender’] above. If we want to create a list of a dictionary’s values instead, we’ll have to access the values with dict ... Why not use a dictionary instead, or use a nested list? Whenever you are thinking: I need to generate variable names, stop. You want a dictionary instead, with your 'variable names' keys in that dictionary. If the names are sequential (numbered starting at …May 3, 2020 ... This Video will help you to understand how to create a list in python • What is List? • How to use List • Assigning multiple values to List ...File Used: file. Method 1: Using Pandas. Here, we have the read_csv () function which helps to read the CSV file by simply creating its object. The column name can be written inside this object to access a particular column, the same as we do in accessing the elements of the array. Pandas library has a function named as tolist () that …Understanding these differences is crucial when choosing the right data structure for your specific use case. Creating Lists. Python offers multiple ways to ... Discussion. 00:00 One way to create lists in Python is using loops, and the most common type of loop is the for loop. You can use a for loop to create a list of elements in three steps. 00:10 Step 1 is instantiate an empty list, step 2 is loop over an iterable or range of elements, and step 3 is to append each element to the end of the list. Values of a: [] Type of a: <class 'list'> Size of a: 0 Python Append to Empty List. In Python, we can create an empty list and then later append items to it, using the Python append() function. Example: In this example, we will first create an empty list in Python using the square brackets and then appended the items to it.Some python adaptations include a high metabolism, the enlargement of organs during feeding and heat sensitive organs. It’s these heat sensitive organs that allow pythons to identi...Yes, you can use the *args (splat) syntax: function_that_needs_strings(*my_list) where my_list can be any iterable; Python will loop over the given object and use each element as a separate argument to the function. See the call expression documentation. There is a keyword-parameter equivalent as well, using two stars:Use the List Comprehension Method to Create a List of Lists in Python Use the for Loop to Create a List of Lists in Python We can have a list of many types in Python, like strings, numbers, and more. Python also allows us to have a list within a list called a nested list or a two-dimensional list. In this tutorial, we will learn how to create ...This will create a 2D list of array, where every row is a unique array of values in each column. If you would like a 2D list of lists, you can modify the above to [df[i].unique().tolist() for i in df.columns] ... Get unique from list as values in Pandas python. 3. Create list from pandas dataframe for distinct values in a column. 1.10. You can call it like list ( [iterable]), so the optional needs to be iterable, which float isn't. iterable may be either a sequence, a container that supports iteration, or an iterator object. The direct definition as list would work …Create a Python list filled with the same string over and over and a number that increases based on a variable. 0. Creating an increasing list in Python. 0. Python List of Lists Incrementing. 1. incrementing list values Python 3. 0. Creating an incremental List. 2. Creating lists of increasing length python. 2.list() Syntax · list() Parameters · list() Return Value · Example 1: Create lists from string, tuple, and list · Example 2: Create lists from set and di...Mar 15, 2012 · I just need to generate a list of pixel locations to process in an image. I use a list because the results of this processing will generate an ordered list that will then be analyzed, pruned, sorted with the result being a list of indices indicating the pixels you "want" in the original list. – We can achieve the same result using list comprehension by: # create a new list using list comprehension square_numbers = [num ** 2 for num in numbers] If we compare the two codes, list comprehension is straightforward and simpler to read and understand. So unless we need to perform complex operations, we can stick to list comprehension.Note: In many real-world scenarios, you'll probably face people calling lists arrays and vice versa, which can be pretty confusing. The main difference, at least in the Python world is that the list is a built-in data type, and the array must be imported from some external module - the numpy and array are probably most notable. Another …Nov 8, 2021 · Python lists are mutable objects meaning that they can be changed. They can also contain duplicate values and be ordered in different ways. Because Python lists are such frequent objects, being able to manipulate them in different ways is a helpful skill to advance in your Python career. The Quick Answer: Use the + Operator This, as the name implies, provides ways to generate combinations of lists. Now that you know how the combinations () function works, let’s see how we can generate all possible combinations of a Python list’s items: for n in range ( len (sample_list) + 1 ): list_combinations += list (combinations(sample_list, n))Method 3: Create a matrix in Python using matrix () function. Use the numpy.matrix () function to create a matrix in Python. We can pass a list of lists or a string representation of the matrix to this function. Example: Let’s create a …. Supporting Material. Transcript. Discussion. 00:00 In this lesson, youSupporting Material. Transcript. Discussion. 00:00 In this less The Quick Answer: append () – appends an object to the end of a list. insert () – inserts an object before a provided index. extend () – append items of iterable objects to end of a list. + operator – concatenate multiple lists together. A highlight of the ways you can add to lists in Python! Apr 13, 2023 ... The list in Python is one of the f Let's go through the script line by line. In the first line, we import the csv module. Then we open the file in the read mode and assign the file handle to the file variable. Next, we work on the opened file using csv.reader (). We only need to specify the first argument, iterable, and we specify the comma as the delimiter.A Python list is a convenient way of storing information altogether rather than individually. As opposed to data types such as int, bool, float, str, a list is a compound data type where you can group values together. In Python, it would be inconvenient to create a new python variable for each data point you collected. Apr 3, 2023 ... The list function in Python creat...
Continue Reading