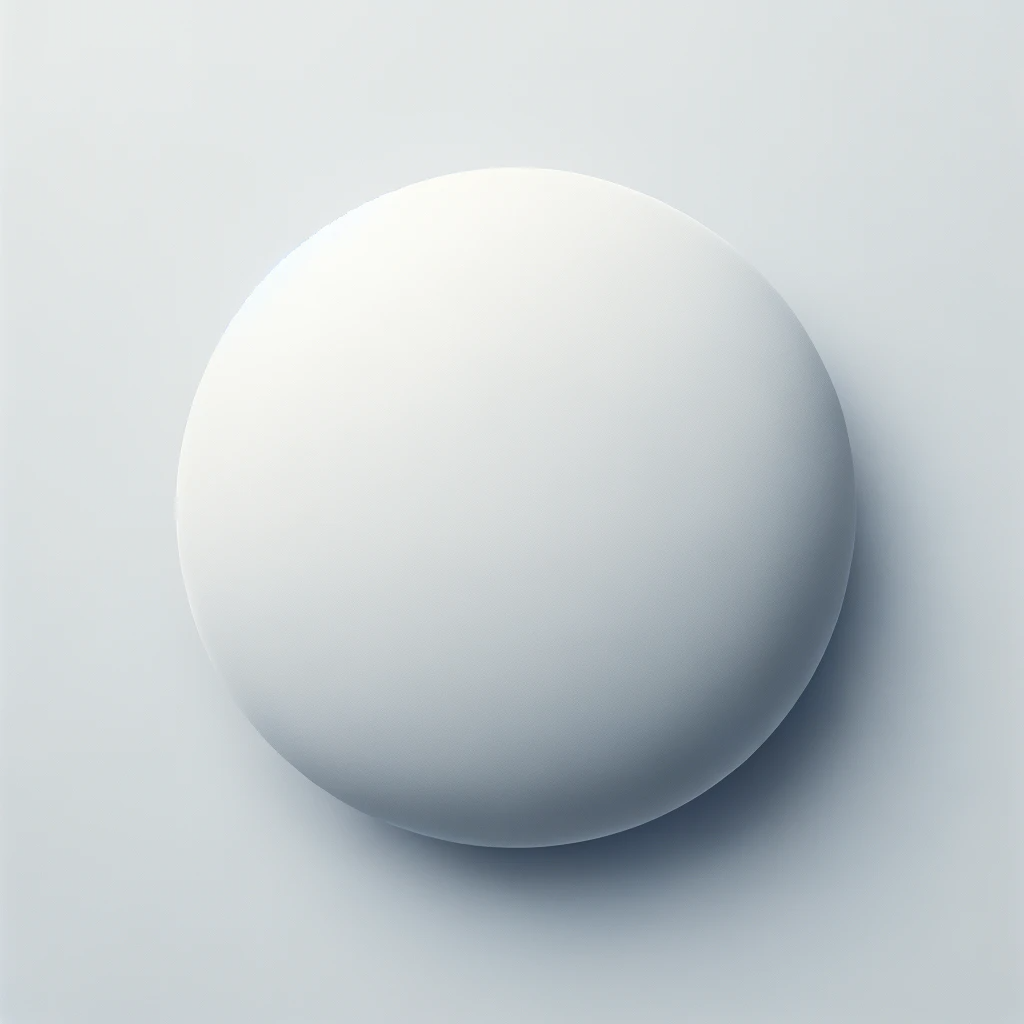
Mar 7, 2024 ... In this tutorial, we will explore ways to Create, Access, Slice, Add, Delete Elements to a Python List along with simple examples.Dec 27, 2023 · Create a List of Tuples Using zip () function. Using the zip () function we can create a list of tuples from n lists. Syntax: list (zip (list1,list2,.,listn) Here, lists are the data (separate lists which are elements like tuples in the list. Example: Python program to create two lists with college ID and name and create a list of tuples using ... In this exercise, you will need to add numbers and strings to the correct lists using the "append" list method. You must add the numbers 1,2, and 3 to the "numbers" list, and the words 'hello' and 'world' to the strings variable. You will also have to fill in the variable second_name with the second name in the names list, using the brackets ...Method 1: - To get the keys using .keys () method and then convert it to list. Method 2: - To create an empty list and then append keys to the list via a loop. You can get the values with this loop as well (use .keys () for just keys and .items () for both keys and values extraction) list_of_keys.append(key)Create a list with initial capacity in Python. # baz. l.append(bar) # qux. This is really slow if you're about to append thousands of elements to your list, as the list will have to be constantly resized to fit the new elements. In Java, you can create an ArrayList with an initial capacity. If you have some idea how big your list will be, this ...You're technically trying to index an uninitialized array. You have to first initialize the outer list with lists before adding items; Python calls this "list comprehension". # Creates a list containing 5 lists, each of 8 items, all set to 0 w, h = 8, 5 Matrix = [[0 for x in range(w)] for y in range(h)] #You can now add items to …Oct 31, 2008 · The append () method adds a single item to the end of the list. The extend () method takes one argument, a list, and appends each of the items of the argument to the original list. (Lists are implemented as classes. “Creating” a list is really instantiating a class. Create a List of Tuples Using zip () function. Using the zip () function we can create a list of tuples from n lists. Syntax: list (zip (list1,list2,.,listn) Here, lists are the data (separate lists which are elements like tuples in the list. Example: Python program to create two lists with college ID and name and create a list of tuples using ...Nice, but some English words truly contain trailing punctuation. For example, the trailing dots in e.g. and Mrs., and the trailing apostrophe in the possessive frogs' (as in frogs' legs) are part of the word, but will be stripped by this algorithm.Handling abbreviations correctly can be roughly achieved by detecting dot-separated …How to Loop Over a List in Python with a List Comprehension. In this section, you’ll learn a unique way of looping over each item in a list. Python comprehensions are a compact way of iterating over a list. Generally, they are used to create lists, either from scratch or by modifying items within the list.Python is a popular programming language known for its simplicity and versatility. Whether you’re a seasoned developer or just starting out, understanding the basics of Python is e...a = ' ' + string.ascii_uppercase. # if start > end, then start from the back. direction = 1 if start < end else -1. # Get the substring of the alphabet: # The `+ direction` makes sure that the end character is inclusive; we. # always need to go one *further*, so when starting from the back, we. # need to substract one.Dec 6, 2023 ... To create a list of Tuples in Python, you can use direct initialization by creating a list of tuples and specifying the tuples within square ...To use lists in a dictionary to create a Pandas DataFrame, we Create a dictionary of lists and then Pass the dictionary to the pd.DataFrame () constructor. Optionally, we can specify the column names for the DataFrame by passing a list of strings to the columns parameter of the pd.DataFrame () constructor. Python3. import pandas as …Dec 3, 2016 · 2. To make this more readable, you can make a simple function: def flatten_list (deep_list: list [list [object]]): return list (chain.from_iterable (deep_list)). The type hinting improves the clarity of what's going on (modern IDEs would interpret this as returning a list [object] type). – Chris Collett. Pseudo-Code. def main(): create an empty list value = getInput() while value isnt zero: add value to the list value = getInput() printOutput(list) def getInput(): prompt the user for a value make sure that the value is an int (convert to int) return the number def printOutput(list): print out the number of input values print out the individual input values …Sep 20, 2010 · Explore Teams Create a free Team. Teams. ... What is the difference between Python's list methods append and extend? 106. Insert some string into given string at ... Program to cyclically rotate an array by one in Python | List Slicing; Python | Check if list contains all unique elements; Python Program to Accessing index and value in list; Python | Print all the common elements of two lists; Python | Cloning or Copying a list; Python | Ways to shuffle a list; How to count unique values inside a listYou can make a shorter list in Python by writing the list elements separated by a comma between square brackets. Running the code squares = [1, 4, 9, 16, ...Python. pal_gen = infinite_palindromes() for i in pal_gen: digits = len(str(i)) pal_gen.send(10 ** (digits)) With this code, you create the generator object and iterate through it. The program only yields a value once a palindrome is found. It uses len () to determine the number of digits in that palindrome.Sep 25, 2022 · Create a List with range function in Python. The range() function returns a sequence of numbers, starting from 0 by default, and increments by 1 (by default), and stops before a specified number.In Python 3, we can combine the range function and list function to create a new list. Range function Syntax Pre-allocating a list of None. Suppose you want to write a function which yields a list of objects, and you know in advance the length n of such list. In python the list supports indexed access in O (1), so it is arguably a good idea to pre-allocate the list and access it with indexes instead of allocating an empty list and using the append ...I just need to generate a list of pixel locations to process in an image. I use a list because the results of this processing will generate an ordered list that will then be analyzed, pruned, sorted with the result being a list of indices indicating the pixels you "want" in the original list. –Aug 4, 2023 ... Using Simple Multiplication · Define the dimensions of the list of lists. Let's say we want to create a list of size n with each sublist ...In today’s digital age, Python has emerged as one of the most popular programming languages. Its versatility and ease of use have made it a top choice for many developers. As a res...Creating a list of lists in python is a little tricky. In this article, we will discuss 4 different ways to create and initialize list of lists. Wrong way to create & initialize a list …Jan 29, 2024 ... The list function creates a list from an iterable object. An iterable may be either a sequence, a container that supports iteration, or an ...However, in this article you’ll only touch on a few of them, mostly for adding or removing elements. First, you need to create a linked list. You can use the following piece of code to do that with deque: Python. >>> from collections import deque >>> deque() deque([]) The code above will create an empty linked list.Python has become one of the most widely used programming languages in the world, and for good reason. It is versatile, easy to learn, and has a vast array of libraries and framewo...Python is a popular programming language known for its simplicity and versatility. Whether you’re a seasoned developer or just starting out, understanding the basics of Python is e...a = ' ' + string.ascii_uppercase. # if start > end, then start from the back. direction = 1 if start < end else -1. # Get the substring of the alphabet: # The `+ direction` makes sure that the end character is inclusive; we. # always need to go one *further*, so when starting from the back, we. # need to substract one.Create a List of Tuples Using zip () function. Using the zip () function we can create a list of tuples from n lists. Syntax: list (zip (list1,list2,.,listn) Here, lists are the data (separate lists which are elements like tuples in the list. Example: Python program to create two lists with college ID and name and create a list of tuples using ...The built-in range function in Python is very useful to generate sequences of numbers in the form of a list. If we provide two parameters in range The first one is starting point, and second one is end point.Dec 27, 2023 · Create a List of Tuples Using zip () function. Using the zip () function we can create a list of tuples from n lists. Syntax: list (zip (list1,list2,.,listn) Here, lists are the data (separate lists which are elements like tuples in the list. Example: Python program to create two lists with college ID and name and create a list of tuples using ... The built-in range function in Python is very useful to generate sequences of numbers in the form of a list. If we provide two parameters in range The first one is starting point, and second one is end point.Jun 3, 2017 · Because you're appending empty_list at each iteration, you're actually creating a structure where all the elements of imp_list are aliases of each other. E.g., if you do imp_list[1].append(4), you will find that imp_list[0] now also has that extra element. So, instead, you should do imp_list.append([]) and make each element of imp_list independent. or, to make it into a list: l=[a+b for pair in itertools.product(list1,list2) for a,b in itertools.permutations(pair,2)] print(l) ... permutations of a lists python. 283. How can I match up permutations of a long list with a shorter list (according to the length of the shorter list)? 2.Using Python's input() function, you can send user input to a list and save it as a string. After that, the input can be processed to generate a list format.Use a For Loop to Make a Python List of the Alphabet. We can use the chr () function to loop over the values from 97 through 122 in order to generate a list of the alphabet in lowercase. The lowercase letters from a …This concise, practical article walks you through a couple of different ways to create a list in Python 3. Table Of Contents. 1 Using square brackets. 2 Using the list() function. 3 Using list comprehension. 4 Initializing an empty list and adding elements later. Using square brackets.The list numbers consists of two lists, each containing five integers. When you use this list of lists to create a NumPy array, the result is an array with two rows and five columns. The function returns the number of rows in the array when you pass this two-dimensional array as an argument in len().These are just a few examples of how you can create a list in Python. Once you have created a list, you can access, modify, and perform various operations on the list using Python’s built-in list methods. Accessing data from Lists. In Python, you can access individual items or a range of items from a list using their index.I have a list of variable length and want to create a checkbox (with python TKinter) for each entry in the list (each entry corresponds to a machine which should be turned on or off with the checkbox -> change the value in the dictionary). print enable {'ID1050': 0, 'ID1106': 0, 'ID1104': 0, 'ID1102': 0} (example, can be any length)Mar 25, 2022 · Create a List of Lists in Python. To create a list of lists in python, you can use the square brackets to store all the inner lists. For instance, if you have 5 lists and you want to create a list of lists from the given lists, you can put them in square brackets as shown in the following python code. The list comprehensions actually are implemented more efficiently than explicit looping (see the dis output for example functions) and the map way has to invoke an ophaque callable object on every iteration, which incurs considerable overhead overhead.. Regardless, [[] for _dummy in xrange(n)] is the right way to do it and none of the tiny (if existent at all) speed …If you want a list you need to explicitly convert that to a list, with the list function like I have shown in the answer. Note 2: We pass number 9 to range function because, range function will generate numbers till …I want to create a very longer list, but ,in python (i don't know in another one), the lists have a limit (24~30, i think), the question is this: Is it ...Oct 10, 2019 · The function itertools.repeat doesn't actually create the list, it just creates an object that can be used to create a list if you wish! Let's try that again, but converting to a list: >>> timeit.timeit('list(itertools.repeat(0, 10))', 'import itertools', number = 1000000) 1.7508119747063233 So if you want a list, use [e] * n. In python, as far as I know, there are at least 3 to 4 ways to create and initialize lists of a given size: Simple loop with append: my_list = [] for i in range(50): my_list.append(0) …Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () function to it, forming a 2D list. The resulting structure is then printed using the `print` statement. Python.python: creating list from string. 14. Reading a list stored in a text file. 13. How do I parse a string representing a nested list into an actual list? 7. python string list to list ast.listeral_eval. 3. Converting a string that represents a list, into an actual list object. See more linked questions.A list is generated in Python programming by putting all of the items (elements) inside square brackets [], separated by commas. It can include an unlimited number of elements of various data types (integer, float, string, etc.). Python Lists can also be created using the built-in list () method. Example.Mar 12, 2024 · Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () function to it, forming a 2D list. The resulting structure is then printed using the `print` statement. Python. Below are the ways by which we can use list() function in Python: To create a list from a string; To create a list from a tuple; To create a list from set and dictionary; Taking user input as a list; Example 1: Using list() to Create a List from a String. In this example, we are using list() function to create a Python list from a …Editor's note: In Python, lists do not have a set capacity, but it is not possible to assign to elements that aren't already present.Answers here show code that creates a list with 10 "dummy" elements to replace later. However, most beginners encountering this problem really just want to build a list by adding elements to it.That should be done using the …Hi. I am trying to create a new dictionary by using an existing list of players for its keys and values from an existing dictionary for its values. The values need to be …Create Lists. Python Basics: Lists and Tuples Martin Breuss 06:33. Mark as Completed. Supporting Material. Transcript. Discussion. 00:00 In this lesson, you’ll learn how you …Jun 29, 2021 ... In this Python list tutorial, you will learn what is a list in Python and how to use list Python. All the concepts of list are disucseed in ...Mar 12, 2024 · Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () function to it, forming a 2D list. The resulting structure is then printed using the `print` statement. Python. Creating Lists in Python. We create lists in Python using square brackets [ ]. To create a list, enclose elements or values in square brackets separated by .... Jun 29, 2021 ... In this Python list tutorial, you will leaCreating 2D List using Naive Method. Here we How to Convert a List to an Array in Python. To convert a list to an array in Python, you can use the array module that comes with Python's standard library. The array module provides a way to create arrays of various types, such as signed integers, floating-point numbers, and even characters.Create a List with list comprehension in Python. List comprehension offers a shorter syntax when we want to create a new list based on the values of an existing list. List comprehension is a sublime way to define and build lists with the help of existing lists. In comparison to normal functions and … 1.Import the re module. 2.Define the input string. 3.Use re.findall Learn three ways to create lists in Python: loops, map(), and list comprehensions. Compare the benefits and drawbacks of each approach and see examples of list …Learn how to create a list in Python using a template and examples. Also, learn how to access items in a list by index, range, or arithmetic operations. Jul 4, 2023 · In Python, list slicing is a common practice and it i...
Continue Reading