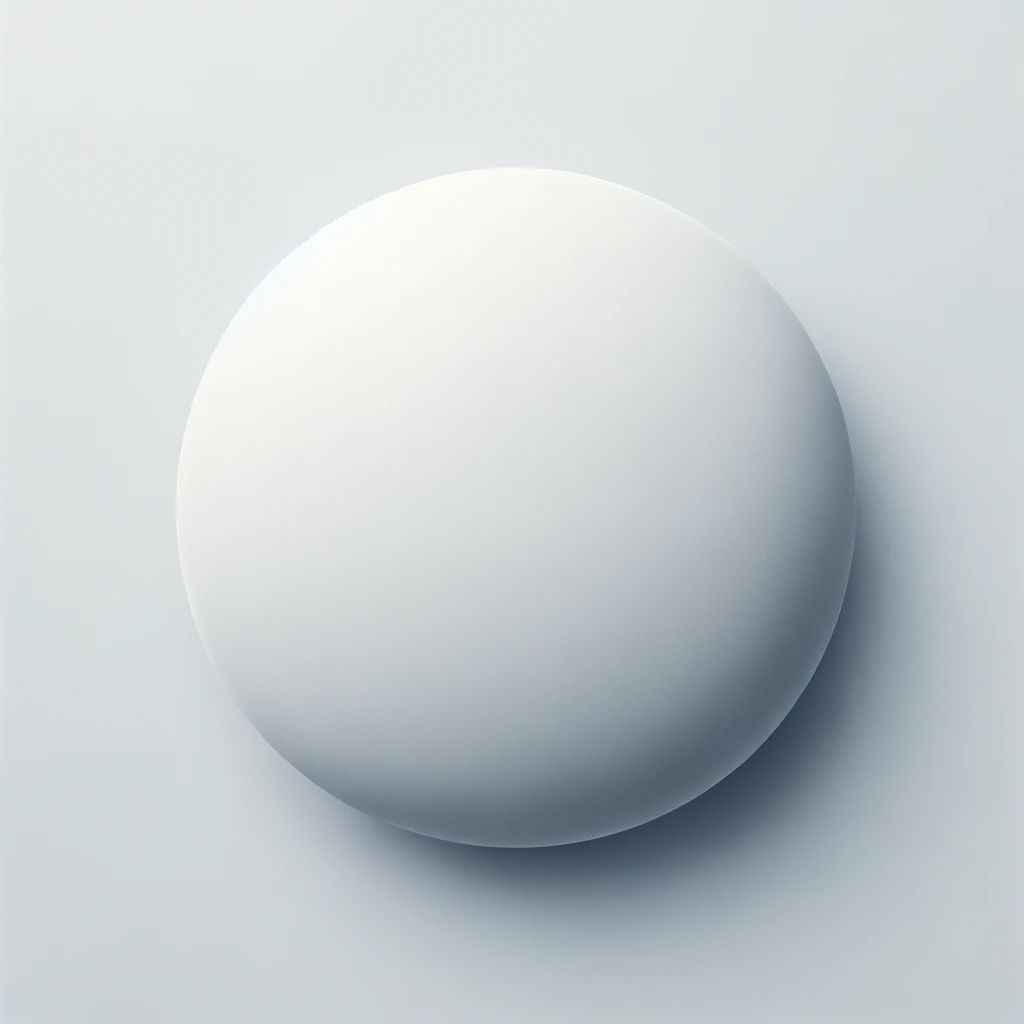
What is Linked List in Python. A linked list is a type of linear data structure similar to arrays. It is a collection of nodes that are linked with each other. A node contains two things first is data and second is a link that connects it with another node. Below is an example of a linked list with four nodes and each node contains character ...Python has become one of the most popular programming languages in recent years. Whether you are a beginner or an experienced developer, there are numerous online courses available...1. A Simple for Loop. Using a Python for loop is one of the simplest methods for iterating over a list or any other sequence (e.g. tuples, sets, or dictionaries ). Python …To create a list in Python, add comma-separated values between braces. For instance, here is a list of names in Python: names = ["Alice", "Bob", "Charlie"] 4. ... Given a list named example.txt with the following contents: This is a test The file contains a bunch of words Bye! W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Method-1: Python select from a list using Indexing. This method involves accessing a specific element in a list by its index, which is the position of the element in the list. The index starts from 0 for the first element and increments by 1 for each subsequent element. # Create a list of countries.3 days ago · List comprehensions provide a concise way to create lists. Common applications are to make new lists where each element is the result of some operations applied to each member of another sequence or iterable, or to create a subsequence of those elements that satisfy a certain condition. For example, assume we want to create a list of squares, like: The core of extensible programming is defining functions. Python allows mandatory and optional arguments, keyword arguments, and even arbitrary argument lists. More about defining functions in Python 3. Python is a programming language that lets you work quickly and integrate systems more effectively. Learn More.list clear () is an in-built function in Python, that is used to delete every element from a list. It can make any list into a null list and should be used with caution. Unmute. ×. It is one of the most basic and known list operations. It is very useful for re-using a list, as you can delete every element from that list.11 May 2023 ... Element: This is the list element or the string character whose lowest index/position will be returned. Start_pos: This specifies the position ...The following code examples show you how to perform actions and implement common scenarios by using the AWS SDK for Python (Boto3) with Amazon S3. Actions are code excerpts from larger programs and must be run in context. While actions show you how to call individual service functions, you can see actions in context in their related scenarios ...Aug 20, 2021 · A list is a data structure in Python that is a mutable, or changeable, ordered sequence of elements. Each element or value that is inside of a list is called an item. Just as strings are defined as characters between quotes, lists are defined by having values between square brackets [ ]. Lists are great to use when you want to work with many ... Mar 1, 2023 · A call stack is an ordered list of functions that are currently being executed. For example, you might call function A, which calls function B, which calls function C. We now have a call stack consisting of A, B, and C. When C raises an exception, Python will look for an exception handler in this call stack, going backward from end to start. Aug 29, 2018 · sample () is an inbuilt function of random module in Python that returns a particular length list of items chosen from the sequence i.e. list, tuple, string or set. Used for random sampling without replacement. Syntax : random.sample (sequence, k) Parameters: sequence: Can be a list, tuple, string, or set. k: An Integer value, it specify the ... Learn everything you need to know about Python lists, one of the most used data structures in Python. See how to create, access, modify, sort, slice, reverse, and …Course. Python Lists (With Examples) List can be seen as a collection: they can hold many variables. List resemble physical lists, they can contain a number of items. A list …Free Download: Get a sample chapter from Python Tricks: ... <iterable> is a collection of objects—for example, a list or tuple. The <statement(s)> in the loop body are denoted by indentation, as with all Python control structures, and are executed once for each item in …What is the List pop method ()? pop () function removes and returns the value at a specific index from a list. It is an inbuilt function of Python. It can be used with and without parameters; without a parameter list pop () returns and removes the last value from the list by default, but when given an index value as a parameter, it only returns ...Some python adaptations include a high metabolism, the enlargement of organs during feeding and heat sensitive organs. It’s these heat sensitive organs that allow pythons to identi...Mar 29, 2022 · Negative Indexing in List. Sometimes, we are interested in the last few elements of a list or maybe we just want to index the list from the opposite end, we can use negative integers. The process of indexing from the opposite end is called Negative Indexing. In negative Indexing, the last element is represented by -1. Example: An exception is an unexpected event that occurs during program execution. For example, divide_by_zero = 7 / 0. The above code causes an exception as it is not possible to divide a number by 0. Let's learn about Python Exceptions in detail.There are two types of loops in Python and these are for and while loops. Both of them work by following the below steps: 1. Check the condition. 2. If True, execute the body of the block under it. And update the iterator/ the value on which the condition is checked. 3. If False, come out of the loop.Mar 1, 2023 · A call stack is an ordered list of functions that are currently being executed. For example, you might call function A, which calls function B, which calls function C. We now have a call stack consisting of A, B, and C. When C raises an exception, Python will look for an exception handler in this call stack, going backward from end to start. Try it out! This tutorial provides a basic Python programmer’s introduction to working with gRPC. By walking through this example you’ll learn how to: Define a service in a .proto file. Generate server and client code using the protocol buffer compiler. Use the Python gRPC API to write a simple client and server for your service.In Python, can use use the range() function to get a sequence of indices to loop through an iterable. You'll often use range() in conjunction with a for loop.. In this tutorial, you'll learn about the different ways in which you can use the range() function – with explicit start and stop indices, custom step size, and negative step size.. Let's get started.Are you a Python developer tired of the hassle of setting up and maintaining a local development environment? Look no further. In this article, we will explore the benefits of swit...Learn how to create, access, modify, sort, and use lists in Python. This tutorial covers the basics and advanced features of lists, such as slicing, copying, …Python List Exercises, Practice and Solution - Contains 280 Python list exercises with solutions for beginners to advanced programmers. These exercises cover various topics such as summing and multiplying items, finding large and small numbers, removing duplicates, checking emptiness, cloning or copying lists, generating 3D arrays, …Learn how to create, access, modify, sort, and use lists in Python. This tutorial covers the basics and advanced features of lists, such as slicing, copying, …The list.extend () method is equivalent to list [len (list):] = iterable. The list.extend () is Python’s built-in function that loops through the provided iterable, appending the elements to the end of the current list. Here we are extending a list with another list’s elements with extend () function. Python3. l = [1, 2, 3] l.extend ( [4, 5 ...In Python, there are two ways to add elements to a list: extend () and append (). However, these two methods serve quite different functions. In append () we add a single element to the end of a list. In extend () we add multiple elements to a list. The supplied element is added as a single item at the end of the initial list by the append ...Python Program to Access Index of a List Using for Loop; Python Program to Flatten a Nested List; Python Program to Slice Lists; Python Program to Iterate Over …In this example, running python program.py -s hello world will produce Concatenated strings: hello world. Example 3: Using an integer for a fixed number of values. import argparse # Initialize the ArgumentParser parser = argparse.ArgumentParser(description="A program to demonstrate nargs with a fixed …Python is one of the most popular programming languages in the world. It is known for its simplicity and readability, making it an excellent choice for beginners who are eager to l...Apr 27, 2021 · 🔹 List and Dictionary Comprehension in Python. A really nice feature of Python that you should know about is list and dictionary comprehension. This is just a way to create lists and dictionaries more compactly. List Comprehension in Python. The syntax used to define list comprehensions usually follows one of these four patterns: 7 Jul 2022 ... We can return the range of elements between two positions in the list. This concept is called slicing. To return the elements in the range, we ... A list is an ordered collection of items. Python uses the square brackets ( []) to indicate a list. The following shows an empty list: empty_list = [] Code language: Python (python) Typically, a list contains one or more items. To separate two items, you use a comma (,). For example: You can also pass in built-in Python functions. For example if you had a list of strings, you can easily create a new list with the length of each string in the list. org_list = ["Hello", "world", "freecodecamp"] fin_list = list(map(len, org_list)) print(fin_list) # [5, 5, 12] How to Use Functions with Multiple Iterables in PythonA list of benchmark fractions include 1/4, 1/3, 1/2, 2/3 and 3/4. Benchmark fractions are common fractions that are used for comparison to other numbers. For example, the benchmark...The groupby () function takes two arguments: (1) the data to group and (2) the function to group it with. Here, lambda x: x [0] tells groupby () to use the first item in each tuple as the grouping key. In the above for statement, groupby returns three (key, group iterator) pairs - once for each unique key.You can use the insert () method to insert an item to a list at a specified index. Each item in a list has an index. The first item has an index of zero (0), the second has an index of one (1), and so on. Here's an example using the insert () method: myList = ['one', 'two', 'three']Python is one of the most popular programming languages in the world, known for its simplicity and versatility. If you’re a beginner looking to improve your coding skills or just w...The Python list() constructor returns a list in Python. In this tutorial, we will learn to use list() in detail with the help of examples. Courses Tutorials Examples . Try Programiz PRO. Course Index Explore Programiz ... Example 3: Create a list from an iterator objectHere's an example: # Joining list elements into a single string. all_fruits = ''.join(fruits) print(all_fruits) In this code snippet, we use an empty string '' as the …In Python, a for loop is used to iterate over sequences such as lists, strings, tuples, etc. languages = ['Swift', 'Python', 'Go'] # access elements of the list one by one for i in languages: print(i) Output. Swift Python Go. In the above example, we have created a list called languages. As the list has 3 elements, the loop iterates 3 times.Clone or Copy a List. When you execute new_List = old_List, you don’t actually have two lists. The assignment just copies the reference to the list, not the actual list. So, both new_List and old_List refer to the same list after the assignment. You can use slicing operator to actually copy the list (also known as a shallow copy).Approach: In this approach, we can create a 3D list using nested for loops. We will start by creating an empty list and then using three for loops to iterate over the dimensions and append the ‘#’ character to the list. Create an empty list lst to hold the 3D list. Loop through the range x to create the first dimension of the 3D list.Dec 17, 2019 · What Is a List in Python? A list is a data structure that's built into Python and holds a collection of items. Lists have a number of important characteristics: List items are enclosed in square brackets, like this [item1, item2, item3]. Lists are ordered – i.e. the items in the list appear in a specific order. This enables us to use an index ... Examples. 1. Sort elements in a list. Following is simple example program where we use sorted () built-in function to sort a list of numbers. We provide this list of numbers as iterable, and not provide key and reverse values as these are optional parameters. Python Program. nums = [2, 8, 1, 6, 3, 7, 4, 9]The syntax of del statement is "del target_list", where target_list can be list, dictionary, item within a list, variable etc. Courses Tutorials Examples . Try Programiz PRO. ... Python del Keyword; Example: Delete an object; Example: Delete variable, list, and dictionary; Example: Remove items from a list; Example: Remove a key:value pair from ... Learn how to create, access and modify lists in Python, one of the four built-in data types for storing collections of data. See examples of list items, list length, list types and list methods. list count () function in Python is a built-in function that lets you count the occurrence of an element in a list. It returns the count of how many times an element is present in a list. It has various applications depending on how you use it. For example: If the count of any element is greater than 1 that means there are duplicate values.A list [/news/how-to-make-a-list-in-python-declare-lists-in-python-example/] is one of the data structures in Python that you can use to store a collection of variables. In some cases, you have to iterate through and perform an operation on the elements of a list. But you can't loop/iterate through a list if it hasMay 15, 2023 · A list can contain different data types and other container objects such as a list, tuple, set, or dictionary. A set can contain only contain immutable objects such as integers, strings, tuples, and floating-point numbers. We can create nested lists in Python. We cannot create nested sets in Python. 7 Jul 2022 ... We can return the range of elements between two positions in the list. This concept is called slicing. To return the elements in the range, we ...14 Sept 2019 ... Accessing the items in a list (and in other iterables like tuples and strings) is a fundamental skill for Python coders, and many Python tools ...W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. List is one of the most frequently used and very versatile data types used in Python. Somewhat similar of characteristics to an array in other programming languages. With the site we could show you some most common use cases with list in python with practical examples. All examples on this page were tested on both python 3 and python 2. Learn how to define, manipulate, and use lists and tuples in Python, two of the most versatile and useful data types. See examples of ordered, mutable, and dynamic …Aug 11, 2023 · How to Create a List in Python. To create a list in Python, write a set of items within square brackets ( []) and separate each item with a comma. Items in a list can be any basic object type found in Python, including integers, strings, floating point values or boolean values. For example, to create a list named “z” that holds the integers ... here if the file does not exist with the mentioned file directory then python will create a same file in the specified directory, and "w" represents write, if you want to read a file then replace "w" with "r" or to append to existing file then "a". newline="" specifies that it removes an extra empty row for every time you create row so to ...Python List Length. Python List Length represents the number of elements in the list. To get the length of list in Python, call the python global function len() with the list passed as argument.. You could also use looping statements, like for loop or while loop, to find the number of elements in a list.But, that would become inefficient, since we have inbuilt …In Python, a for loop is used to iterate over sequences such as lists, strings, tuples, etc. languages = ['Swift', 'Python', 'Go'] # access elements of the list one by one for i in languages: print(i) Output. Swift Python Go. In the above example, we have created a list called languages. As the list has 3 elements, the loop iterates 3 times. Here is the logical equivalent code in Python. This function takes a Python object and optional parameters for slicing and returns the start, stop, step, and slice length for the requested slice. def py_slice_get_indices_ex(obj, start=None, stop=None, step=None): length = len(obj) if step is None: step = 1. Aug 29, 2018 · sample () is an inbuilt function of random module in Python that returns a particular length list of items chosen from the sequence i.e. list, tuple, string or set. Used for random sampling without replacement. Syntax : random.sample (sequence, k) Parameters: sequence: Can be a list, tuple, string, or set. k: An Integer value, it specify the ... list clear () is an in-built function in Python, that is used to delete every element from a list. It can make any list into a null list and should be used with caution. Unmute. ×. It is one of the most basic and known list operations. It is very useful for re-using a list, as you can delete every element from that list.You’ll learn, for example, how to append two lists, combine lists sequentially, combine lists without duplicates, and more. Being able to work with Python lists is an incredibly important skill. ... We can also use a Python list comprehension to combine two lists in Python. This approach uses list comprehensions a little unconventionally: we ...22 Nov 2008 ... @erhesto You judged the answer as not correct, because the author used references as an example to fill a list? First, no one is requiring ...Dec 17, 2019 · What Is a List in Python? A list is a data structure that's built into Python and holds a collection of items. Lists have a number of important characteristics: List items are enclosed in square brackets, like this [item1, item2, item3]. Lists are ordered – i.e. the items in the list appear in a specific order. This enables us to use an index ... The for loop in Python is an iterating function. If you have a sequence object like a list, you can use the for loop to iterate over the items contained within the list. The functionality of the for loop isn’t very different from what you see in multiple other programming languages.Dec 21, 2023 · Here are some examples and use-cases of list append() function in Python. Append to list in Python using List.append() Method. List.append() method is used to add an element to the end of a list in Python or append a list in Python, modifying the list in place. For example: `my_list.append(5)` adds the element `5` to the end of the list `my_list`. Microsoft Outlook's Visual Basic for Applications macro programming language enables you to add a form to an email message that can collect data from its recipient. For example, yo...The Python list() constructor returns a list in Python. In this tutorial, we will learn to use list() in detail with the help of examples. Courses Tutorials Examples . Try Programiz PRO. Course Index Explore Programiz ... Example 3: Create a list from an iterator object Python offers the following list functions: sort (): Sorts the list in ascending order. type (list): It returns the class type of an object. append (): Adds a single element to a list. extend (): Adds multiple elements to a list. index (): Returns the first appearance of the specified value. In this example, we created a two-dimensional list called data containing nested lists. The DataFrame() function converts the 2-D list to a DataFrame. Each nested list behaves like a row of data in the DataFrame. The columns argument provides a name to each column of the DataFrame. Note: We can also create a DataFrame using NumPy array in a ...Apr 9, 2021 · Python list is an ordered sequence of items. In this article you will learn the different methods of creating a list, adding, modifying, and deleting elements in the list. Also, learn how to iterate the list and access the elements in the list in detail. Nested Lists and List Comprehension are also discussed in detail with examples. Open-source programming languages, incredibly valuable, are not well accounted for in economic statistics. Gross domestic product, perhaps the most commonly used statistic in the w...1.If the input array has length 0 or 1, return the array as it is already sorted. 2.Choose the first element of the array as the pivot element. 3.Create two empty lists, left and right. 4.For each element in the array except for the pivot: a. If the element is smaller than the pivot, add it to the left list.Inside flatten_extend(), you first create a new empty list called flat_list.You’ll use this list to store the flattened data when you extract it from matrix.Then you start a loop to iterate over the inner, or nested, lists from matrix.In this example, you use the name row to represent the current nested list.. In every iteration, you use .extend() to add the … The Python list() constructor returns a list in Python. In this tutorial, we will learn to use list() in detail with the help of examples. Courses Tutorials Examples . Inside flatten_extend(), you first create a new empty list called flat_list.You’ll use this list to store the flattened data when you extract it from matrix.Then you start a loop to iterate over the inner, or nested, lists from matrix.In this example, you use the name row to represent the current nested list.. In every iteration, you use .extend() to add the …In the above example, the personal_info is a list of tuples where the get_age function is defined as a key function. Here, the list is sorted based on the age (i.e., second element) of each tuple. Sort Example With Both Parameters We can use both key and reverse parameters for sorting the list. For example, words = ['python', 'is', 'awesome ...Python List max() Method Example. Lets look at some examples to find max element in Python list: Example 1: In this example, we are going to find the maximum integer value in the list. For that, we have to make a list of some random integers and then use the Python list max() function to find the maximum value in the list. Let’s implement ...If you are a Python programmer, it is quite likely that you have experience in shell scripting. It is not uncommon to face a task that seems trivial to solve with a shell command. ...For programmers, this is a blockbuster announcement in the world of data science. Hadley Wickham is the most important developer for the programming language R. Wes McKinney is amo.... Use the random.choices () function to select multiple random items f W3Schools offers free online tutorials, references and exercises 13 Jul 2023 ... get() function. You can access an item in the list by referring to its index number. Python list index starts from 0. The following are examples ...Dec 27, 2023 · Below are some examples which depict the use of list comprehensions rather than the traditional approach to iterate through iterable: Python List Comprehension using If-else. In the example, we are checking that from 0 to 7 if the number is even then insert Even Number to the list else insert Odd Number to the list. zip() in Python Examples Python zip() with lists. In Pyt In Python, we can create a dictionary of lists using the following 9 methods. Moreover, for each method, we will also illustrate an example. Using dictionary literals. Using dict () & zip () methods. Using a dictionary comprehension. Using defaultdict () … 2 Mar 2020 ... Python allows updating single or multiple ...
Continue Reading